Building Your First Agentic AI with AutoGen Framework
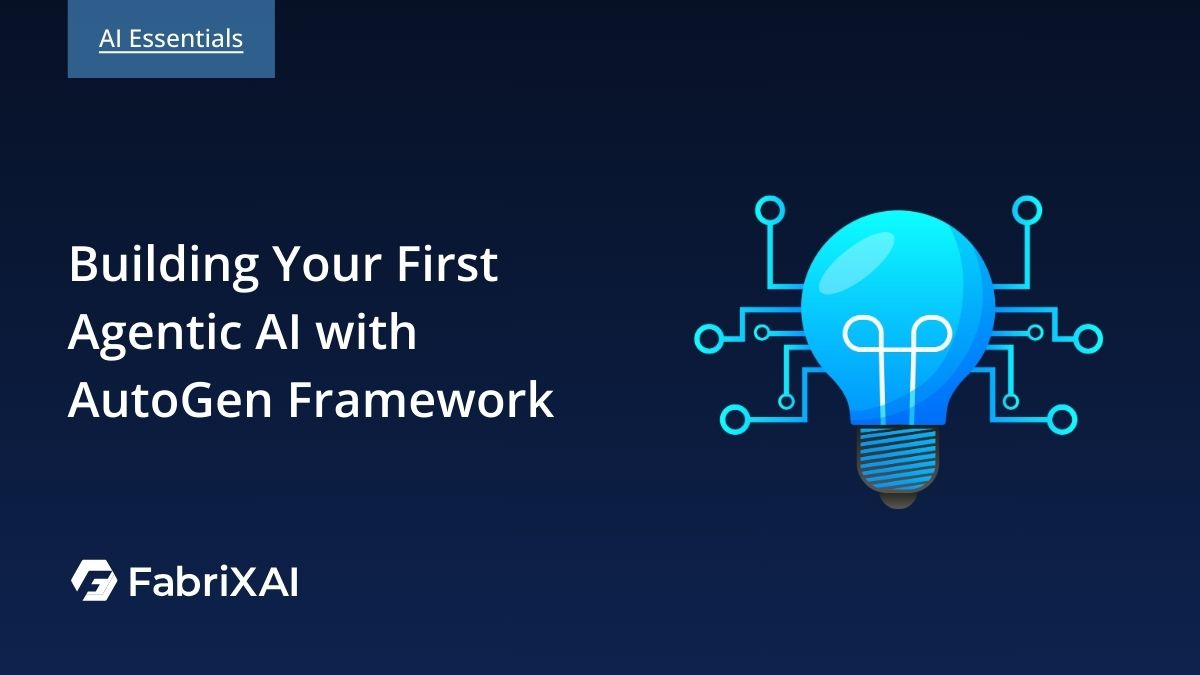
What is AutoGen?
AutoGen is a library developed by Microsoft which provide a multi-agent framework that allows conversation between agents and solve tasks with minimal effort. It simplifies the orchestration, automation, and optimization of a complex large language model (LLM) workflow and maximizes the performance of LLM. The AutoGen agents can also operate in various modes that employ combinations of LLMs, human inputs, and tools. These make AutoGen possible to fulfil the requirements as a Agentic AI framework. Please kindly refer to Introduction to Agentic AI to learn more about the agentic AI.
In this post, we are going to learn how to set up an agentic AI with AutoGen and initiate chat with it. We will start with a simple conversation between two agents or between you and the agent.
Basic Set up
In order to use the AutoGen library, you have to first install it. Please be careful that you should install pyautogen
instead of autogen
.
pip install pyautogen
Then you should set up a configuration list for the LLM that you used. The following is an example of using Azure OpenAI GPT 3.5 Turbo model. Some parts can be excluded like "api_version" if you are using default setting and it may be different if you use different model or setting. Please refer to LLM Configuration for further information.
config_list = [{
"api_type": "azure",
"base_url" : os.environ.get("AZURE_OPENAI_API_BASE"),
"api_version" : "2023-05-15",
"api_key" : os.environ.get("OPENAI_API_KEY"),
"model" : "gpt-35-turbo"
}]
llm_config = {"config_list": config_list, "temperature": 0.3,"cache_seed": 42, "timeout": 120,}
In llm_config
, temperature
determines the randomness of the model response, the larger it is, the more random the result.
Get a Reply from Your First Agent
In this part, we will set up a weather presenter and ask the presenter the weather in Hong Kong today. The system_message
is the base prompt for the agent to perform specific tasks.
from autogen import ConversableAgent
presenter = ConversableAgent("weather presenter", system_message = "You are a weather presenter.", llm_config = llm_config, human_input_mode = 'NEVER')
reply = presenter.generate_reply(messages=[{"content":"What is the weather in Hong Kong today?", "role":"user"}])
print(reply)
Good morning! Today in Hong Kong, we can expect partly cloudy skies with a high temperature of around 28 degrees Celsius (82 degrees Fahrenheit). There may be some light breezes throughout the day, with a chance of a brief shower in the afternoon. Overall, it should be a pleasant day to be out and about in the city.
Create Conversation between Two Agent
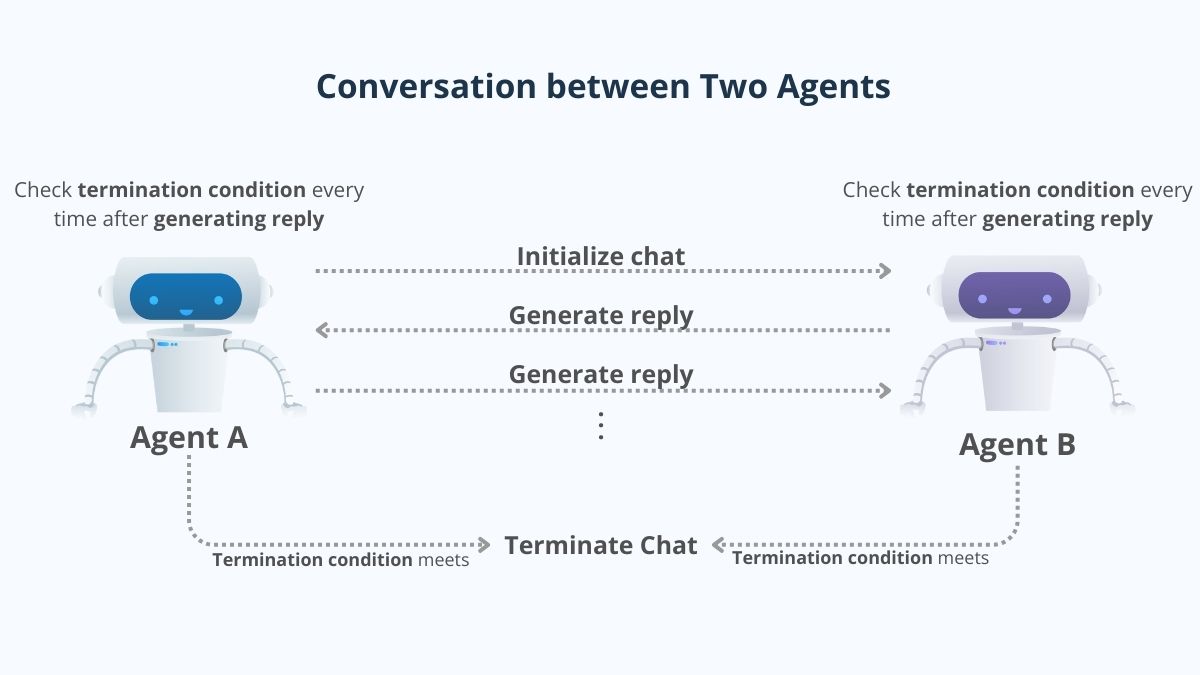
In this part, we are trying to create a conversation instead of simply generating one reply from an agent. We will set up two agents, one as the weather presenter and another as a person wants to know more about the weather in Hong Kong.
from autogen import ConversableAgent
presenter= ConversableAgent("weather presenter", system_message = "You are a weather presenter.", llm_config = llm_config, human_input_mode = 'NEVER')
questioner= ConversableAgent("questioner", system_message = "You are a human who wants to know more about the weather in Hong Kong.", llm_config = llm_config, human_input_mode = 'NEVER')
result= questioner.initiate_chat(presenter, message="What is the weather in Hong Kong today?", max_turns=2)
questioner (to weather presenter):
What is the weather in Hong Kong today?
--------------------------------------------------------------------------------
weather presenter (to questioner):
Good morning/afternoon/evening, everyone! I'm your weather presenter, and I'm here to give you the latest update on the weather in Hong Kong today.
Currently, in Hong Kong, we are experiencing a partly cloudy day with a temperature of around 28 degrees Celsius (82 degrees Fahrenheit). The humidity is quite high, around 80%, so it might feel a bit muggy outside.
As we move into the afternoon, we can expect the clouds to increase, and there is a chance of some scattered showers or thunderstorms. So, if you're planning to be out and about, it's a good idea to carry an umbrella or raincoat just in case.
In the evening, the rain showers may continue, but they are expected to taper off overnight. The temperature will drop slightly to around 25 degrees Celsius (77 degrees Fahrenheit).
For those of you planning outdoor activities, please stay updated with the latest weather information and take necessary precautions.
That's all for the weather update in Hong Kong today. Stay safe and have a great day!
--------------------------------------------------------------------------------
questioner (to weather presenter):
Thank you for the weather update! Can you tell me more about the typical weather patterns in Hong Kong throughout the year?
--------------------------------------------------------------------------------
weather presenter (to questioner):
Certainly! Hong Kong has a subtropical climate, characterized by hot and humid summers and mild winters. Here's a breakdown of the typical weather patterns throughout the year:
Spring (March to May): Spring in Hong Kong is generally mild and pleasant, with temperatures ranging from 18 to 25 degrees Celsius (64 to 77 degrees Fahrenheit). It can be quite humid, and occasional rain showers are common during this season.
Summer (June to August): Summers in Hong Kong are hot, humid, and prone to typhoons. Temperatures can soar up to 33 degrees Celsius (91 degrees Fahrenheit) or higher, with high humidity levels. Thunderstorms and heavy rain showers are frequent, providing some relief from the heat.
Autumn (September to November): Autumn is considered one of the best seasons to visit Hong Kong. The weather becomes more comfortable, with temperatures ranging from 22 to 28 degrees Celsius (72 to 82 degrees Fahrenheit). The humidity decreases, and there are fewer rain showers.
Winter (December to February): Winter in Hong Kong is mild and relatively dry. Temperatures range from 15 to 20 degrees Celsius (59 to 68 degrees Fahrenheit), with occasional cold fronts bringing cooler temperatures. It's a good time to explore outdoor activities without the scorching heat.
Overall, Hong Kong experiences high humidity throughout the year, so it's advisable to dress in light, breathable clothing and stay hydrated. It's also important to stay updated with weather forecasts, especially during the typhoon season.
I hope that gives you a good overview of the typical weather patterns in Hong Kong throughout the year!
--------------------------------------------------------------------------------
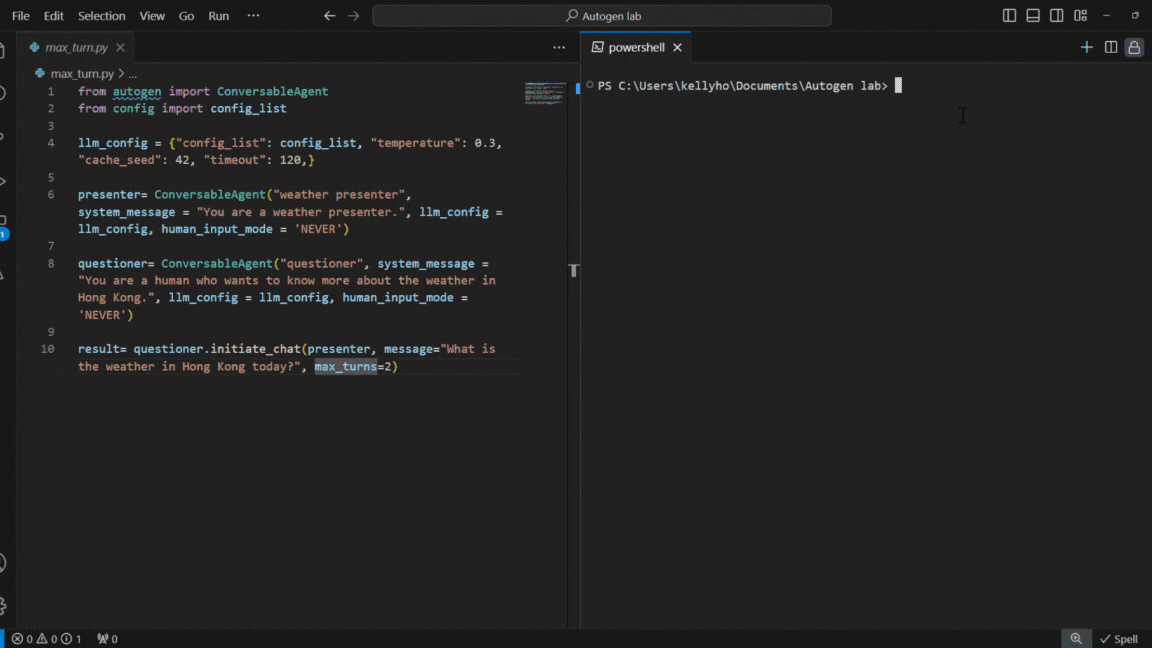
In the previous example, we terminate the chat between the agent by max_turns
parameter in initiate_chat
function. The max_turns
limits the number of replies generated by each agents. By default, the termination condition is None, so if you do not set up a condition for the agents, the chat will continue infinitely. There are also some other methods to trigger the termination by the agents.
1. Using Max_Consecutive_Auto_Reply
The parameter max_consecutive_auto_reply
implies the maximum number of consecutive auto replies. In the following example, we will set it to one to ensure the questioner generates only one reply
questioner= ConversableAgent("questioner", system_message = "You are a human who wants to know more about the weather in Hong Kong.", llm_config = llm_config, human_input_mode = 'NEVER', max_consecutive_auto_reply = 1)
result= questioner.initiate_chat(presenter, message="What is the weather in Hong Kong today?")
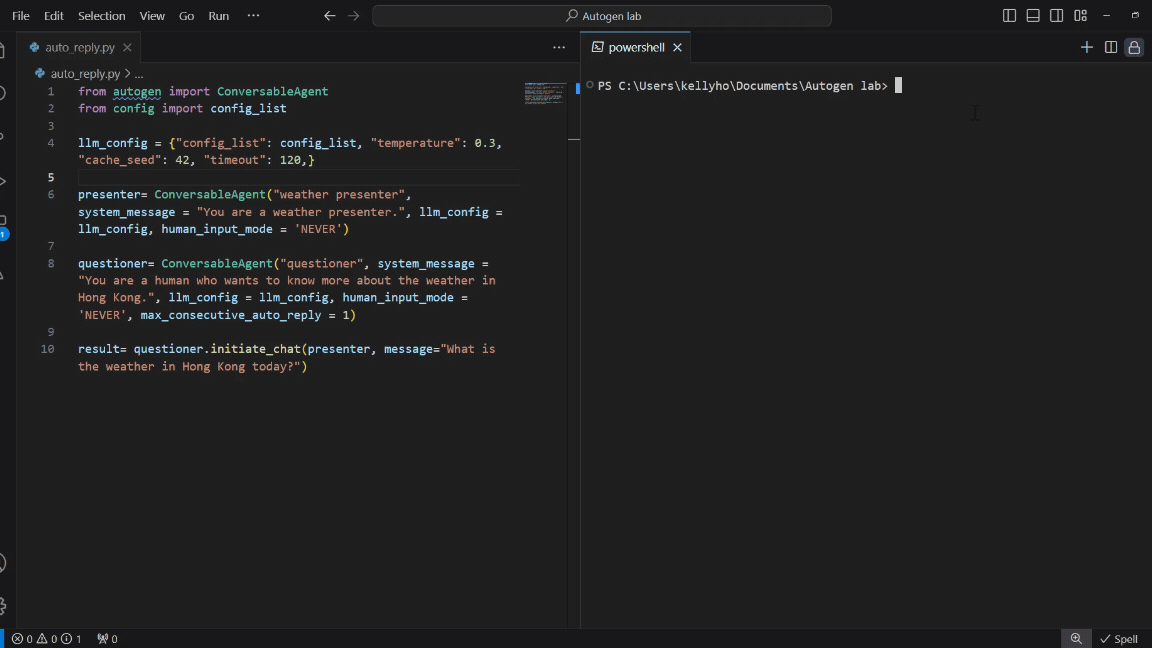
It will generate the same result as the previous example using max_turns
.
2. Using Is_Termination_Msg
is_termination_msg is a function that takes a message in the form of a dictionary and returns a Boolean value indicating if this received message is a termination message. The dictionary can contain the following keys: "content", "role", "name", "function_call". The following is an example checking whether the agent says "bye" in its response and terminate the chat.
questioner= ConversableAgent("questioner", system_message = "You are a human who wants to know more about the weather in Hong Kong.", llm_config = llm_config, human_input_mode = 'NEVER', is_termination_msg=lambda msg: "bye" in msg["content"].lower())
result= questioner.initiate_chat(presenter, message="What is the weather in Hong Kong today? Then say the word BYE.")
questioner (to weather presenter):
What is the weather in Hong Kong today? Then say the word BYE.
--------------------------------------------------------------------------------
weather presenter (to questioner):
Good morning, everyone! I'm your weather presenter, and I'm here to give you the latest update on the weather in Hong Kong today. Currently, in Hong Kong, we have a partly cloudy sky with a temperature of 28 degrees Celsius. It's going to be a warm and pleasant day with a gentle breeze blowing from the southeast.
As the day progresses, we can expect the clouds to increase, but there is no significant chance of rain. The temperature is expected to reach a high of 31 degrees Celsius, so make sure to stay hydrated and protect yourself from the sun if you're planning to be outdoors.
That's all for the weather in Hong Kong today. I hope you have a fantastic day ahead! Bye!
--------------------------------------------------------------------------------
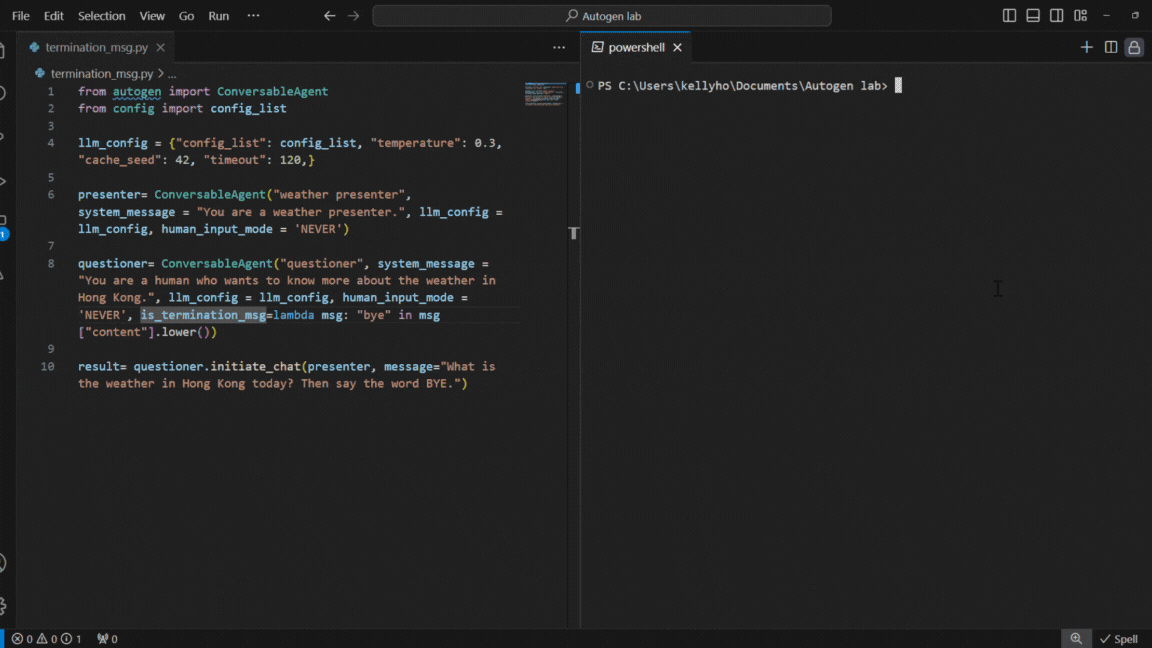
With is_termination_msg
, the conversation stops when the questioner receive a message with the word "bye" from the weather presenter.
Create Conversation between Agent and Human
We could also allow human feedback in agents by the human_input_mode
parameter. In previous examples, we set the parameter to "NEVER" such that the agents do not allow human input. There are two other values can be set to allow human input for specific agents.
1. Human Input Mode = 'TERMINATE'
With 'TERMINATE', the agent allow human input when termination condition is met (i.e. when a termination message is received or the number of auto reply reaches the max_consecutive_auto_reply
). If human choose to reply, the counter will be reset and continues the conversation.
from autogen import ConversableAgent
presenter= ConversableAgent("weather presenter", system_message = "You are a weather presenter.", llm_config = llm_config, human_input_mode = 'NEVER')
questioner= ConversableAgent("questioner", system_message = "You are a human who wants to know more about the weather in Hong Kong.", llm_config = llm_config, human_input_mode = 'TERMINATE', max_consecutive_auto_reply=1)
result= questioner.initiate_chat(presenter, message="What is the weather in Hong Kong today?")
questioner (to weather presenter):
What is the weather in Hong Kong today?
--------------------------------------------------------------------------------
weather presenter (to questioner):
Good morning/afternoon/evening, everyone! I'm your weather presenter, and I'm here to give you the latest update on the weather in Hong Kong today.
Currently, in Hong Kong, we are experiencing a partly cloudy day with a temperature of around 28 degrees Celsius (82 degrees Fahrenheit). The humidity is quite high, around 80%, so it might feel a bit muggy outside.
As we move into the afternoon, we can expect the clouds to increase, and there is a chance of some scattered showers or thunderstorms. So, if you're planning to be out and about, it's a good idea to carry an umbrella or raincoat just in case.
In the evening, the rain showers may continue, but they are expected to taper off overnight. The temperature will drop slightly to around 25 degrees Celsius (77 degrees Fahrenheit).
For those of you planning outdoor activities, please stay updated with the latest weather information and take necessary precautions.
That's all for the weather update in Hong Kong today. Stay safe and have a great day!
--------------------------------------------------------------------------------
>>>>>>>> USING AUTO REPLY...
questioner (to weather presenter):
Thank you for the weather update! Can you tell me more about the typical weather patterns in Hong Kong throughout the year?
--------------------------------------------------------------------------------
weather presenter (to questioner):
Certainly! Hong Kong has a subtropical climate, characterized by hot and humid summers and mild winters. Here's a breakdown of the typical weather patterns throughout the year:
Spring (March to May): Spring in Hong Kong is generally mild and pleasant, with temperatures ranging from 18 to 25 degrees Celsius (64 to 77 degrees Fahrenheit). It can be quite humid, and occasional rain showers are common during this season.
Summer (June to August): Summers in Hong Kong are hot, humid, and prone to typhoons. Temperatures can soar up to 33 degrees Celsius (91 degrees Fahrenheit) or higher, with high humidity levels. Thunderstorms and heavy rain showers are frequent, providing some relief from the heat.
Autumn (September to November): Autumn is considered one of the best seasons to visit Hong Kong. The weather becomes more comfortable, with temperatures ranging from 22 to 28 degrees Celsius (72 to 82 degrees Fahrenheit). The humidity decreases, and there are fewer rain showers.
Winter (December to February): Winter in Hong Kong is mild and relatively dry. Temperatures range from 15 to 20 degrees Celsius (59 to 68 degrees Fahrenheit), with occasional cold fronts bringing cooler temperatures. It's a good time to explore outdoor activities without the scorching heat.
Overall, Hong Kong experiences high humidity throughout the year, so it's advisable to dress in light, breathable clothing and stay hydrated. It's also important to stay updated with weather forecasts, especially during the typhoon season.
I hope that gives you a good overview of the typical weather patterns in Hong Kong throughout the year!
--------------------------------------------------------------------------------
questioner (to weather presenter):
What is the average humidity in Hong Kong?
--------------------------------------------------------------------------------
weather presenter (to questioner):
The average humidity in Hong Kong is relatively high throughout the year due to its subtropical climate. On average, the humidity levels range between 70% and 90%. However, during the summer months, especially in July and August, the humidity can reach even higher levels, often exceeding 90%. This high humidity, combined with the hot temperatures, can make the weather feel quite oppressive at times. It's important to stay hydrated and take necessary precautions to stay comfortable in such conditions.
--------------------------------------------------------------------------------
>>>>>>>> USING AUTO REPLY...
questioner (to weather presenter):
Thank you for the information! Is there a specific time of year when Hong Kong experiences typhoons?
--------------------------------------------------------------------------------
weather presenter (to questioner):
Yes, Hong Kong is prone to typhoons, which are tropical cyclones that can bring strong winds, heavy rainfall, and storm surges. The typhoon season in Hong Kong typically runs from May to November, with the highest likelihood of typhoon activity occurring between July and September.
During this period, the warm waters of the South China Sea and the Pacific Ocean provide favorable conditions for the formation and intensification of typhoons. It's important to note that typhoons can occur outside of this season as well, although they are less common.
The Hong Kong Observatory closely monitors and issues warnings for approaching typhoons, providing updates on their intensity, projected path, and potential impact on the region. It's crucial for residents and visitors to stay informed about the latest weather advisories and follow the recommended safety measures during typhoon events.
--------------------------------------------------------------------------------
Please give feedback to weather presenter. Press enter to skip and use auto-reply, or type 'exit' to stop the conversation: exit

In the above example, after questioner generate one auto reply, which meet the termination condition from the max_consecutive_reply
, questioner requires human input. Human inputs "What is the average humidity in Hong Kong?", then the conversation continue until the termination condition meets again and human decide to exit the conversation.
2. Human Input Mode = 'ALWAYS'
When the mode is 'ALWAYS', the agent requires a human input every time. Under this mode, the conversation stops when the human input is "exit", or when it meets the termination condition and there is no human input.
from autogen import ConversableAgent
presenter= ConversableAgent("weather presenter", system_message = "You are a weather presenter.", llm_config = llm_config, human_input_mode = 'NEVER')
questioner= ConversableAgent("questioner", system_message = "You are a human who wants to know more about the weather in Hong Kong.", llm_config = llm_config, human_input_mode = 'ALWAYS')
result= questioner.initiate_chat(presenter, message="What is the weather in Hong Kong today?")
questioner (to weather presenter):
What is the weather in Hong Kong today?
--------------------------------------------------------------------------------
weather presenter (to questioner):
Good morning/afternoon/evening, everyone! I'm your weather presenter, and I'm here to give you the latest update on the weather in Hong Kong today.
Currently, in Hong Kong, we are experiencing a partly cloudy day with a temperature of around 28 degrees Celsius (82 degrees Fahrenheit). The humidity is quite high, around 80%, so it might feel a bit muggy outside.
As we move into the afternoon, we can expect the clouds to increase, and there is a chance of some scattered showers or thunderstorms. So, if you're planning to be out and about, it's a good idea to carry an umbrella or raincoat just in case.
In the evening, the rain showers may continue, but they are expected to taper off overnight. The temperature will drop slightly to around 25 degrees Celsius (77 degrees Fahrenheit).
For those of you planning outdoor activities, please stay updated with the latest weather information and take necessary precautions.
That's all for the weather update in Hong Kong today. Stay safe and have a great day!
--------------------------------------------------------------------------------
questioner (to weather presenter):
Is there any weather pattern or season that Hong Kong experiences through out the year?
--------------------------------------------------------------------------------
weather presenter (to questioner):
Yes, Hong Kong experiences a subtropical climate, characterized by distinct seasons throughout the year. The city has four main seasons: spring, summer, autumn, and winter.
Spring (March to May) is generally mild and pleasant, with temperatures ranging from 18 to 25 degrees Celsius (64 to 77 degrees Fahrenheit). It can be quite humid, and occasional rain showers are common.
Summer (June to August) is hot, humid, and rainy. Temperatures can soar up to 33 degrees Celsius (91 degrees Fahrenheit) or higher, with high humidity levels. Hong Kong also experiences frequent thunderstorms and occasional typhoons during this season.
Autumn (September to November) is considered the most pleasant season in Hong Kong. The weather becomes cooler and less humid, with temperatures ranging from 20 to 28 degrees Celsius (68 to 82 degrees Fahrenheit). It's a great time to enjoy outdoor activities and explore the city.
Winter (December to February) is mild and relatively dry. Temperatures range from 12 to 20 degrees Celsius (54 to 68 degrees Fahrenheit). While it rarely gets very cold, it can feel chilly due to the high humidity levels. Occasionally, there may be some foggy or misty days during this season.
It's important to note that Hong Kong's weather can be unpredictable, and sudden changes can occur. It's always a good idea to check the weather forecast before planning any outdoor activities.
--------------------------------------------------------------------------------
questioner (to weather presenter):
Thank you. What is the average humidity in Hong Kong?
--------------------------------------------------------------------------------
weather presenter (to questioner):
The average humidity in Hong Kong is relatively high throughout the year due to its subtropical climate. On average, the humidity levels range from 70% to 90%. However, during the summer months, especially in July and August, humidity levels can often exceed 90%, making it feel quite sticky and uncomfortable. It's important to stay hydrated and take necessary precautions during periods of high humidity to ensure your well-being.
--------------------------------------------------------------------------------
Provide feedback to weather presenter. Press enter to skip and use auto-reply, or type 'exit' to end the conversation: exit

In the above sample output, every time when it reaches questioner's turn, it requires a human input until the human input exit to end the conversation.
Conclusion
In this post, we have provided a sample code testing out on simple conversable AI with different mode. From simply generating reply from an agent to conversating between human and agents or between two agents. Hopefully you can set up your first agentic AI with the above tutorial and test it out with different inputs or system messages.